Before I go ahead and discuss the three simple rules for mastering Git and Github, please consider completing the following tasks:
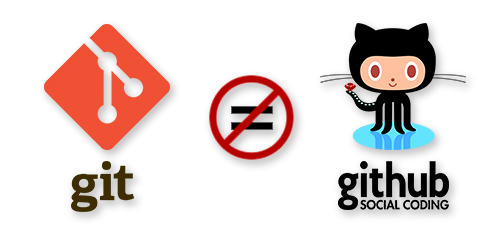
And now, without much further ado, the three simple rules to master Git and Github while learning how to code…
- Rule #1: Create a Git repository for every new project
- Rule #2: Create a new branch for every new feature
- Rule #3: Use Pull Requests to merge code to Master
Even if you are working on small and simple projects, and even if you are working alone, following those three rules every time you code will make you a Git and GitHub master user very quickly.
Rule #1: Create a Git repository for every new project
This first rule is quite straightforward, but making a habit out of it is very important. Every time you start working on something new — your portfolio, a learning project, a solution to a coding challenge, and so on — you should create a new Git repository and push it to GitHub.
Having a dedicated repo is the first step to being able to use version control for every line of code you write. Using version control is how you will work once you join a company and start working on real-world projects. Learn this early and make it a habit.
Quick Note: if using the terminal becomes a hassle and makes you less likely to use Git for all your projects, consider using the Github Desktop app.
Rule #2: Create a new branch for every new feature
Let’s say you are working on your portfolio and you want to build a new “Contact me” section/component. Create a dedicated branch for this new feature, give it a meaningful name (e.g. contact-me-section), and commit all the code to that specific branch.
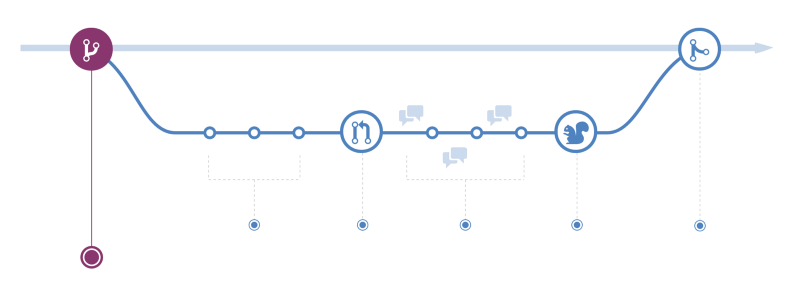
If you don’t know what branches are, go back to the Github Flow reading that I recommended before.
Working with branches allows you and your team members to work on different features in a parallel way while keeping the specific code for each feature isolated from the rest. This makes it harder for unstable code to get merged into the main code base.
Even if you are the only person on your team, getting used to using feature branches will make the Github Flow process a breeze once you join a real job.
Rule #3: Use Pull Requests to merge code to Master
Every repository starts with a master branch by default. You should never push changes directly to the master branch. Instead, you should use feature branches as described above, and open a new Pull Request to merge the feature branch code with the master branch code.
In a real job, someone will look at your Pull Request and do a code review before approving it. GitHub will even run automated tests to your code and let you know if there is an issue with it. You will also be notified if there is any merge conflict between your code and the code in the master branch. This can happen, for example, if another developer pushed a change to the master branch that affects a file that you also modified.
After your code has been reviewed, tested, and approved, your reviewer will give you thumbs up for you to merge the Pull Request, or they will directly merge your pull request.
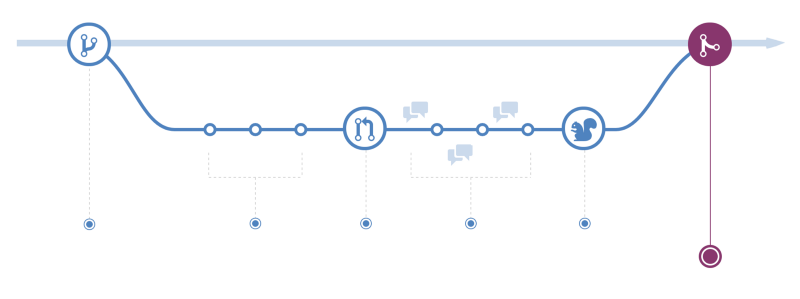
Even if you are working alone, get used to creating Pull Requests as a way to merge your changes to the master branch. This, by the way, is the basic workflow used by almost every open source project. If you ever contribute to one (you should!), understanding this three rules will make it really easy for you to get your contribution accepted without any problem.
If you are still confused, just start slow and keep the three rules in mind. Don’t try to think about “How” to do things yet and focus on “What” to do and “Why” it’s important for now.
Once the “What” and the “Why” are clear, you can figure out the “How” when the times comes to do things. Once you have repeated this process 2–3 times, it will become really easy and natural for you.